7.2.1 Develop the Code for the Insert Button Event Handler
In Section 6.3.3.3 in Chapter 6, we gave a detailed discussion of dynamic data query using the PreparedStatement object method. Refer to that section to get more details about that method.
In this section, we will use that object to perform a dynamic faculty member insertion into the Faculty Table in our sample database.
Open the Insert button click event handler and enter the code shown in Figure 7.2. Let’s have a closer look at this piece of code to see how it works.
A. Some local variables and objects are declared first, which include a local integer variable, numInsert, which is used to hold the returned number of inserted rows as the data insert action is performed, and a byte array, fImage, which is used to hold the selected faculty image to be inserted into the database later.
B. Prior to performing a data insertion, one needs to make sure that all TextFields that contain the seven pieces of new faculty information are filled. To do that, a user-defined method, chkFaculty(), is called to check all pieces of information to make sure that this inser-tion is valid. A warning message will be displayed if any field is empty.
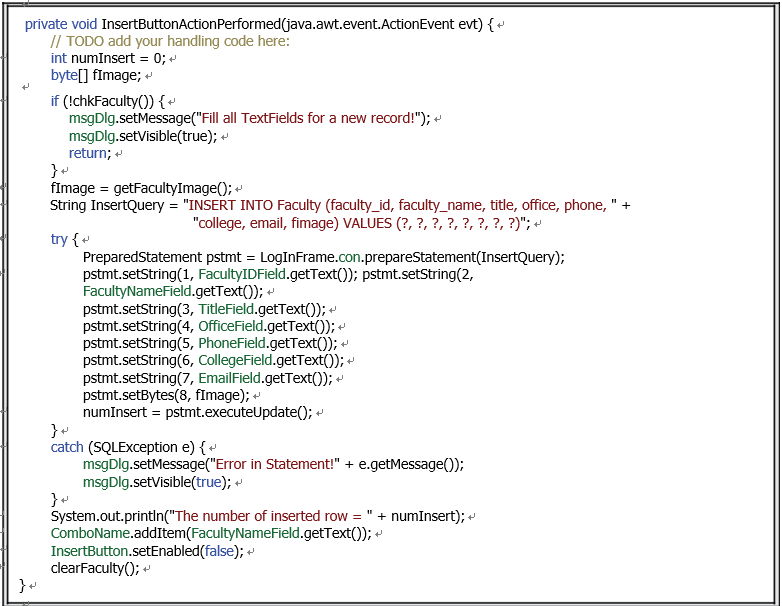
FIGURE 7.2 The added code for the Insert button click event handler.
C. Another user-defined method, getFacultyImage(), is executed to select and obtain a selected faculty image to be inserted into the Faculty Table in our sample database.
D. An insert query string is created with eight positional dynamic parameters, which are associated with the eight pieces of inserted faculty information. One point to be noted is that the order of these parameters must be identical to the order of columns defined in the Faculty Table. Otherwise, an exception may occur when this insertion is performed.
E. A try-catch block is used to initialize and execute the data insertion action. First, a PreparedStatement instance is created using the Connection object that is located at the LogInFrame class with the insert query string as the argument.
F. The setString() method is used to initialize the seven pieces of inserted faculty infor-mation, which are obtained from the seven text fields entered by the user as the project runs. Also, the setBytes() method must be used to set the faculty image column, fim-age, to insert a new selected faculty image into the database.
G. The data insertion function is performed by calling the executeUpdate() method. The run result of this method, which is an integer that equals the number of rows that have been inserted into the database, is assigned to the local variable numInsert.
H. The catch block is used to track and collect any possible exception encountered when this data insertion is executed.
I. The run result is printed out for debugging purposes.
J. The new inserted faculty name is attached to the Faculty Name combo box to enable users to validate this data insertion later.
K. After data insertion, the Insert button must be disabled to avoid any possible duplicated inser-tion operation. To do that, a system method, setEnable(), with a false argument is used.
L. Finally, another user-defined method, clearFaculty(), is called to clean up all pieces of inserted information to make it ready for a validation of this insertion later.
Before we can build and run the project to test the data insertion function, we should first Figure out how to check and validate this data insertion. First let’s take care of the data checking to make sure that all pieces of new inserted faculty information are valid prior to this insertion.